WebRTC 화상회의 서버 구축
WebRTC 화상회의 서버 구축
musma.github.io
WebRTC(Web Real-Time Communication)
- 브라우저와 모바일 애플리케이션에서 실시간 커뮤니케이션을 가능하게 하는 기술
- 음성, 영상, 데이터 채널을 사용할 수 있으며, 다양한 애플리케이션에서 응용할 수 있다.
- P2P(Peer-to-Peer) 연결을 통해 데이터를 전송하므로, 중간 서버를 거치지 않아 지연시간이 적고, 성능이 뛰어나다.
주요 구성 요소
1. 시그널링(Signaling)
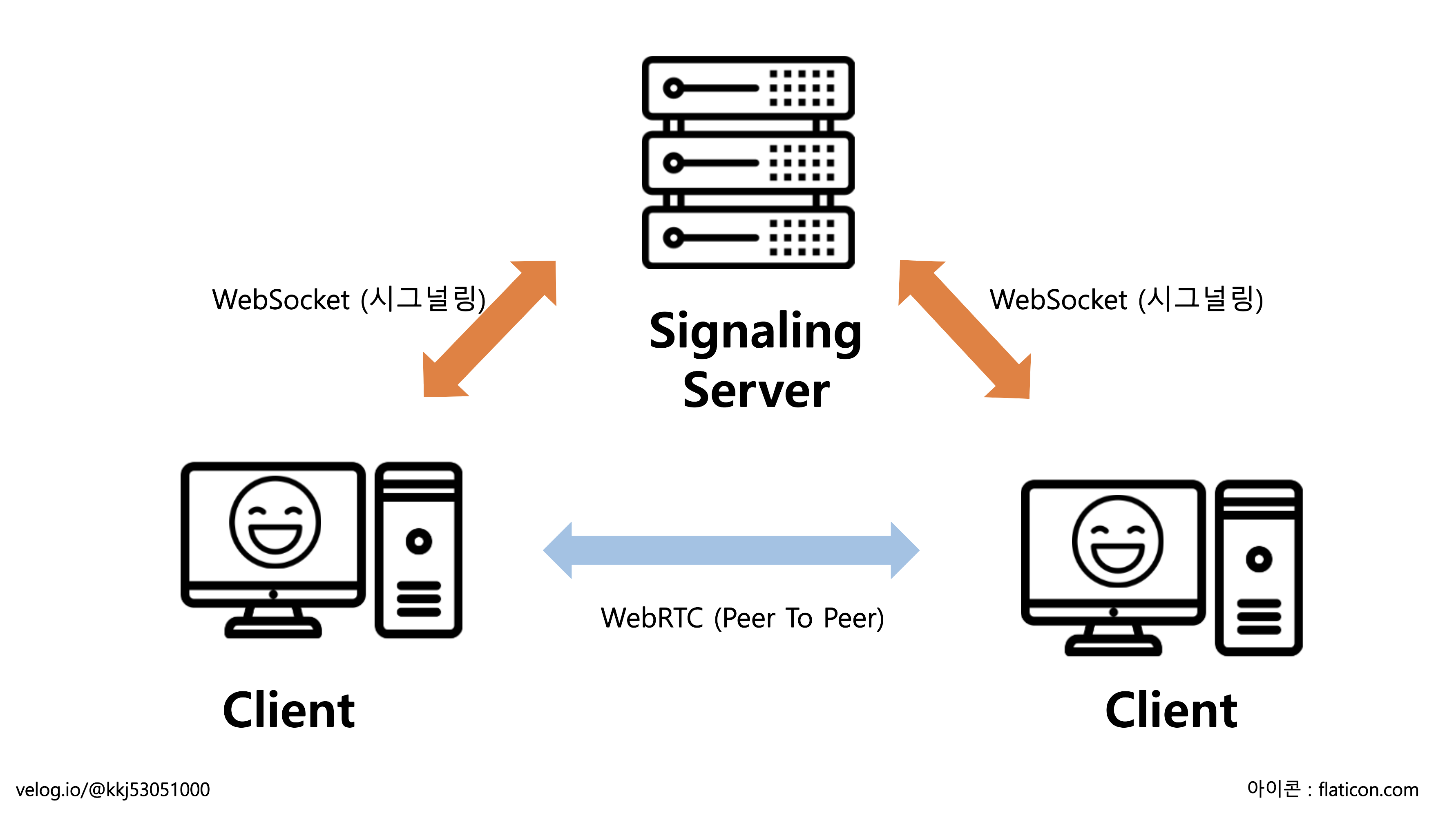
- WebRTC 자체는 시그널링 프로토콜을 정의하지 않는다.
- 시그널링은 두 피어(peer) 간 연결을 설정하고 관리하기 위한 메커니즘
- WebSocket, HTTP, XMPP 등 다양한 프로토콜을 사용할 수 있다.
- 정보 교환 요소는 SDP, ICE가 있다.
SDP (Session Description Protocol)
연결을 설정하기 위한 메타데이터 (예: 코덱 정보, 미디어 타입 등)
ICE (Interactive Connectivity Establishment) candidates
피어 간의 연결을 설정하는 데 필요한 네트워크 정보 (예: IP 주소, 포트 등)
2. SDP (Session Description Protocol)
- 세션 초기화를 위해 사용되는 포맷
- 미디어 타입, 코덱, 네트워크 정보 등을 포함한다.
ex.
v=0
o=- 4611737351243714426 2 IN IP4 127.0.0.1
s=-
t=0 0
a=group:BUNDLE audio video
a=msid-semantic: WMS
m=audio 9 UDP/TLS/RTP/SAVPF 111 103 9 0 8 106 105 13 126
c=IN IP4 0.0.0.0
a=rtcp:9 IN IP4 0.0.0.0
a=ice-ufrag:EfF5
a=ice-pwd:2B4flSDzI3TxO7ZtDiUuzLfV
a=ice-options:trickle
a=fingerprint:sha-256 12:34:56:78:90:AB:CD:EF:12:34:56:78:90:AB:CD:EF:12:34:56:78:90:AB:CD:EF:12:34:56:78:90:AB:CD:EF
a=setup:actpass
a=mid:audio
a=sendrecv
a=rtcp-mux
a=rtpmap:111 opus/48000/2
a=rtcp-fb:111 transport-cc
a=fmtp:111 minptime=10;useinbandfec=1
3. ICE (Interactive Connectivity Establishment)
- 피어 간의 연결을 설정하는 프로토콜
- STUN (Session Traversal Utilities for NAT) 및 TURN (Traversal Using Relays around NAT) 서버를 사용하여 NAT(Network Address Translation)와 방화벽을 통해 연결할 수 있도록 돕는다.
- 후보지 (candidates)를 수집하고 교환하여 최적의 연결 경로를 찾는다.
WebRTC 연결 과정 예시
1. 시그널링 서버에 연결
- 두 클라이언트가 시그널링 서버를 통해 초기 정보를 교환
2. SDP 교환
- 각 클라이언트는 `RTCPeerConnection` 객체를 생성하고, 자신의 로컬 SDP를 생성
- 이 SDP는 시그널링 서버를 통해 상대 클라이언트에 전달
3. ICE 후보지 교환
- 각 클라이언트는 자신의 ICE 후보지를 수집하여 상대 클라이언트에 전달
- 상대 클라이언트는 이 후보지를 이용해 P2P 연결을 설정
4. 미디어 스트림 추가
- 연결이 설정되면, 각 클라이언트는 `getUserMedia`를 통해 미디어 스트림(예: 오디오, 비디오)을 추가
ex. javascript
// 시그널링 서버 연결 (WebSocket 사용 예시)
const signalingServer = new WebSocket('wss://example.com/signaling');
// RTCPeerConnection 생성
const configuration = {
iceServers: [{ urls: 'stun:stun.example.com' }]
};
const peerConnection = new RTCPeerConnection(configuration);
// ICE 후보지 처리
peerConnection.onicecandidate = event => {
if (event.candidate) {
signalingServer.send(JSON.stringify({ type: 'ice-candidate', candidate: event.candidate }));
}
};
// 원격 스트림 처리
peerConnection.ontrack = event => {
const remoteStream = event.streams[0];
// 원격 스트림을 비디오 요소에 추가
document.getElementById('remoteVideo').srcObject = remoteStream;
};
// 로컬 미디어 스트림 추가
navigator.mediaDevices.getUserMedia({ video: true, audio: true })
.then(stream => {
// 로컬 비디오 요소에 스트림 추가
document.getElementById('localVideo').srcObject = stream;
// 로컬 스트림을 RTCPeerConnection에 추가
stream.getTracks().forEach(track => peerConnection.addTrack(track, stream));
});
// SDP 생성 및 시그널링 서버로 전송
peerConnection.createOffer()
.then(offer => peerConnection.setLocalDescription(offer))
.then(() => {
signalingServer.send(JSON.stringify({ type: 'offer', sdp: peerConnection.localDescription }));
});
// 시그널링 서버로부터 메시지 수신 처리
signalingServer.onmessage = event => {
const message = JSON.parse(event.data);
if (message.type === 'offer') {
peerConnection.setRemoteDescription(new RTCSessionDescription(message.sdp))
.then(() => peerConnection.createAnswer())
.then(answer => peerConnection.setLocalDescription(answer))
.then(() => {
signalingServer.send(JSON.stringify({ type: 'answer', sdp: peerConnection.localDescription }));
});
} else if (message.type === 'answer') {
peerConnection.setRemoteDescription(new RTCSessionDescription(message.sdp));
} else if (message.type === 'ice-candidate') {
peerConnection.addIceCandidate(new RTCIceCandidate(message.candidate));
}
};
결론
WebRTC는 실시간 통신 기술로, 다양한 응용 프로그램에 활용될 수 있다. 시그널링, SDP, ICE 등의 개념을 이해하고 올바르게 구현하는 것이 중요하다.
WebRTC는 고성능의 실시간 커뮤니케이션 솔루션을 제공하여, 애플리케이션도 가능하다.
'CS' 카테고리의 다른 글
OSI 7계층 모델 (0) | 2024.06.17 |
---|---|
모놀리식 vs. 마이크로서비스 아키텍처 (0) | 2024.05.30 |
네트워크 프로토콜과 통신 개념 (0) | 2024.05.17 |
소켓(Socket) 통신 (0) | 2024.05.16 |
UI/UX 용어 (0) | 2024.05.09 |